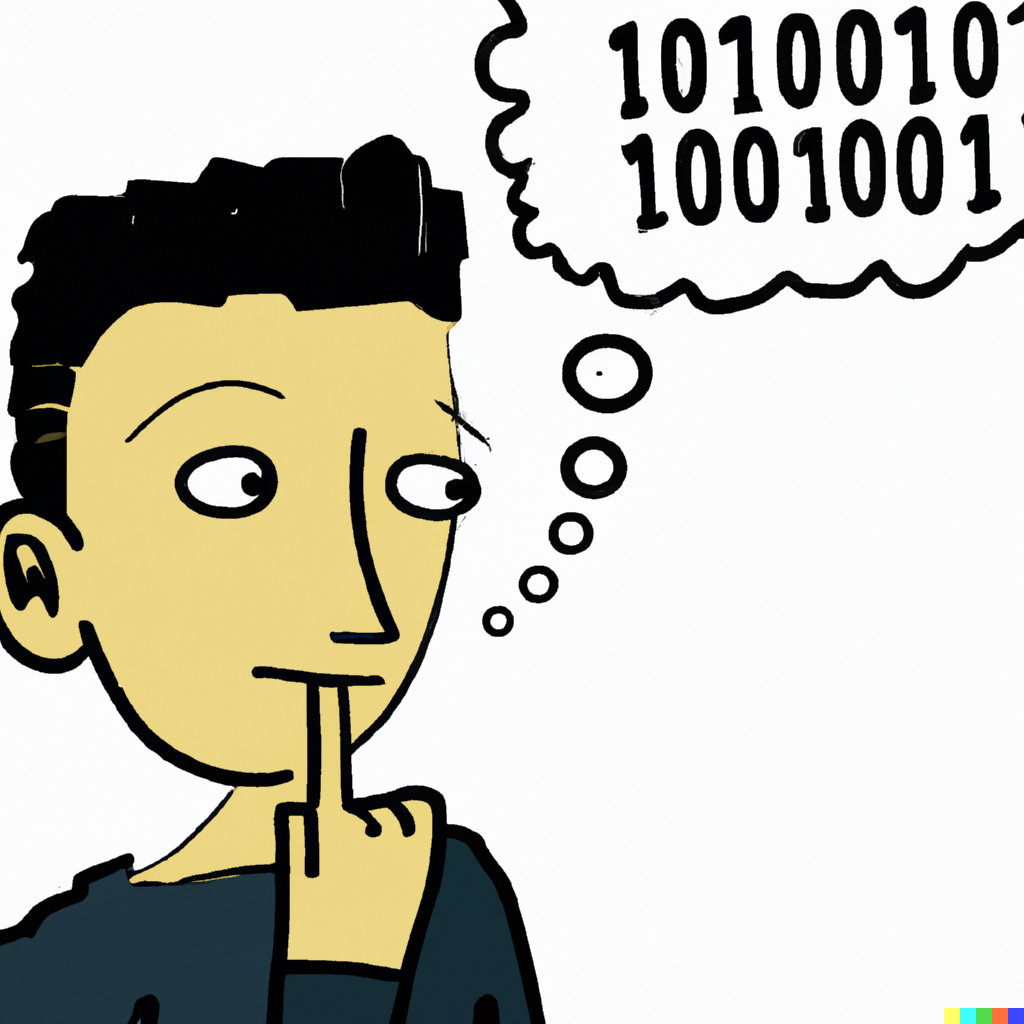
Imagine if I gave you a list of items and asked whether it contained a particular element. If it were, say, a list of ingredients, you would probably have no choice but to read every single entry until you found the target item. For instance, does the following list contain “salt”?
TOMATO CONCENTRATE FROM RED RIPE TOMATOES, DISTILLED VINEGAR, HIGH FRUCTOSE CORN SYRUP, CORN SYRUP, SALT, SPICE, ONION POWDER, NATURAL FLAVORING
If we start at the beginning of the list, we might get lucky, and salt could be the first item in the list. In that case, we could stop right away. Or, we could get unlucky, and it could be the last item in the list, or not appear in the list at all. Thus, in the worst-case scenario, we would have to go through every item in the list once.
A task like this is something that a computer can perform very efficiently. If the list is very large, a computer could search for a target element much faster than a human could. But how does a computer know how to perform these kinds of tasks? The computer is following a list of step-by-step instructions. The technical term for such a set of instructions is an algorithm. An algorithm could be very simple. In the example above, where we are searching for a specific ingredient in a list, we could write the instructions in plain English like so:
Go through each element in the list one by one. If you come across the target element, stop, and conclude that the list contains the element. If you go through the entire list and do not find the element, conclude that the list does not contain the target element.
We could write this in a format that is a little bit closer to computer code by translating it into the raw steps and translating some of the terms into common coding terms, as such:
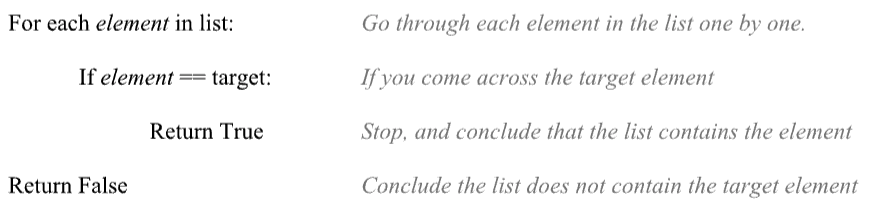
The algorithm written above is not in any particular language, but it does contain all of the steps required to produce the correct answer in every case. This is crucially important for an algorithm—it has to give the correct answer in all cases. Sometimes this means you have to be explicit with including certain steps to a computer that might seem obvious to a human. For instance, we need to clarify in the script above that if the item is not found we return “false”, which might be a step that is easy to forget, since it seems obvious.
Computers are great at following these specific instructions, which is one of the things that makes them powerful.
Learning algorithms and practicing solving problems gives you practical coding experience, because you can start with simple problems that can be solved with only the most basic coding commands and tools. It’s a great way to get started writing your own small programs. Once you’ve learned a little bit and written your own code, it becomes much easier to read other code.
Say you want to do a textual analysis of a book you have on your computer. You can easily google this and find a program someone has already written. If you know how to read the code, it will be very easy to use someone else’s code and quickly debug it if problems arise. If you don’t have any experience reading code, you might well struggle for a hours to solve a problem that could be something as simple as a missing parentheses! Learning to read and understand code—even on a basic level—opens up a whole world of possibilities!
Are you interested in learning more about algorithms or getting into coding? Here are some resources I recommend:
For algorithms and python, come to the Graduate Center’s Python User Group (PUG) meetings.
For practicing algorithms, check out leetcode.com. You can create a free account and solve algorithmic problems in your browser. There are thousands of problems on the site, ranging from very simple to incredibly complex. They also have some free learning resources available.
To learn to code, a great resource is https://www.freecodecamp.org/learn.
Finally, look for part 2 of this blog post at the beginning of the Spring semester!